IMPROVE YOUR ONLINE REPUTATION
Analyze, generate, monitor and share online reviews to improve your company’s reputation and attract new clients.
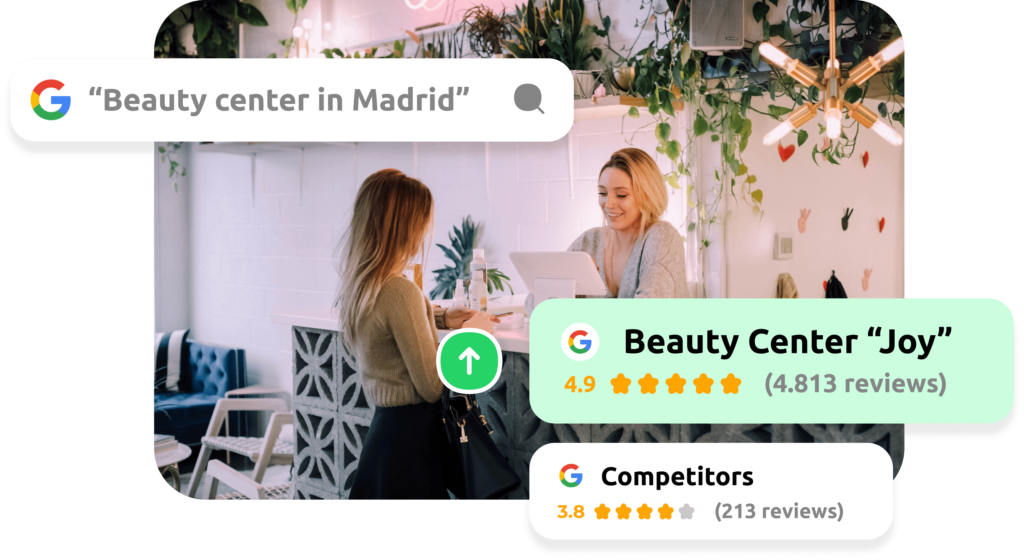
USED BY +XXX LOCAL BUSINESSES WORLDWIDE
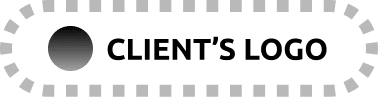
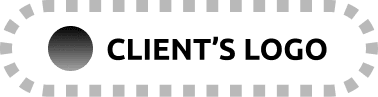
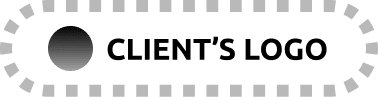
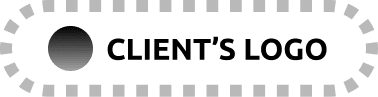
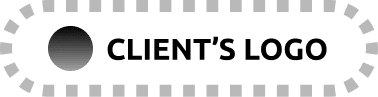
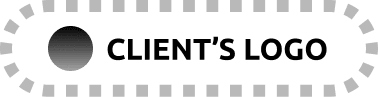
GET REVIEWS
GET NEW POSITIVE REVIEWS, AVOID THE NEGATIVE ONES
Get new reviews by inviting your customers to review you via customizable e-mail or SMS, or on-site via QR code.
Protect your reputation with an optional system that captures dissatisfied customers, keeping negative feedback private.
Choose on which platforms you want to increase the number of positive reviews.
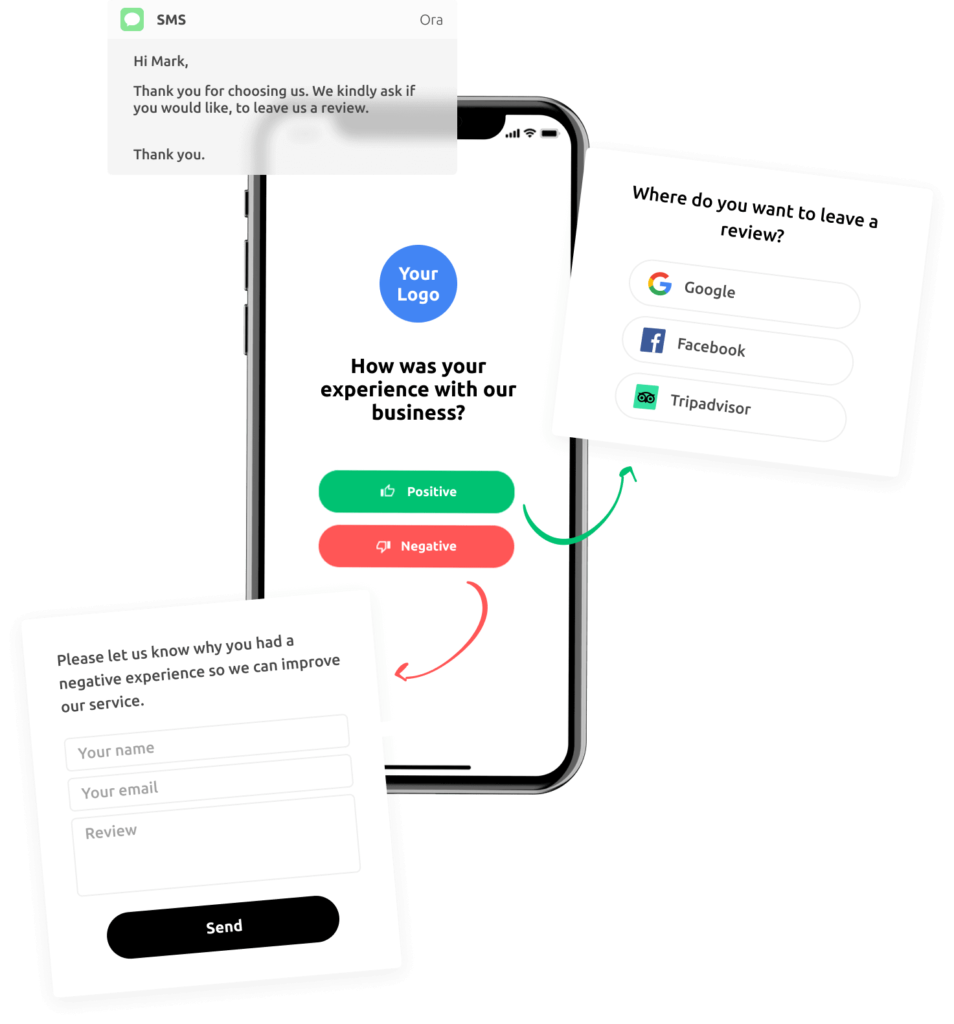
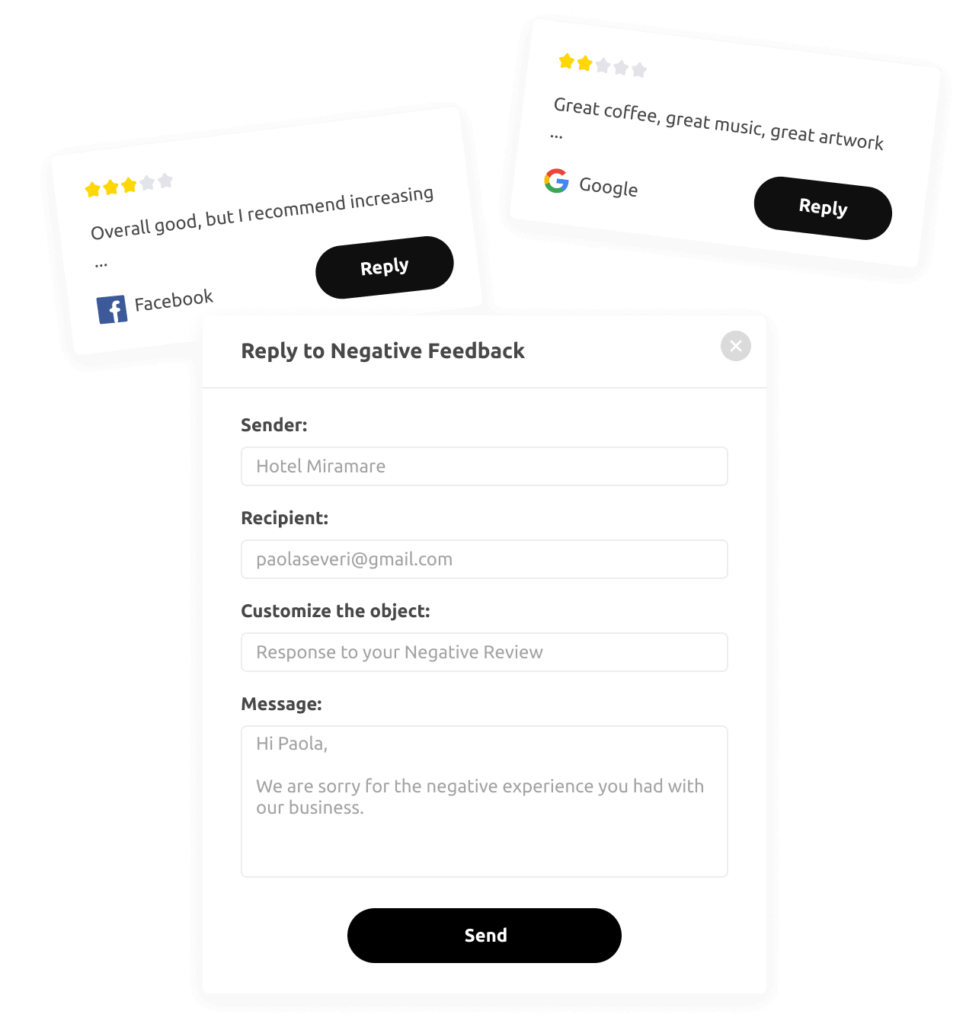
MONITOR AND REPLY
MONITOR AND REPLY TO ALL THE REVIEWS FROM ONE PLACE.
View all reviews received in the various related review platforms, day by day.
Be sure to respond to all reviews you receive to show closeness to your customers.
Respond to private negative feedback to regain your customers’ trust, and figure out how to improve.
SHARE REVIEWS
SHOW YOUR BEST REVIEWS ON YOUR WEBSITE AND SOCIAL MEDIA CHANNELS.
Create a carousel or feed of your best reviews and easily post them on your site.
Share the best reviews on your Facebook or Instagram page via customizable templates.
Schedule the posting of reviews on social to create your own review-based marketing strategy.
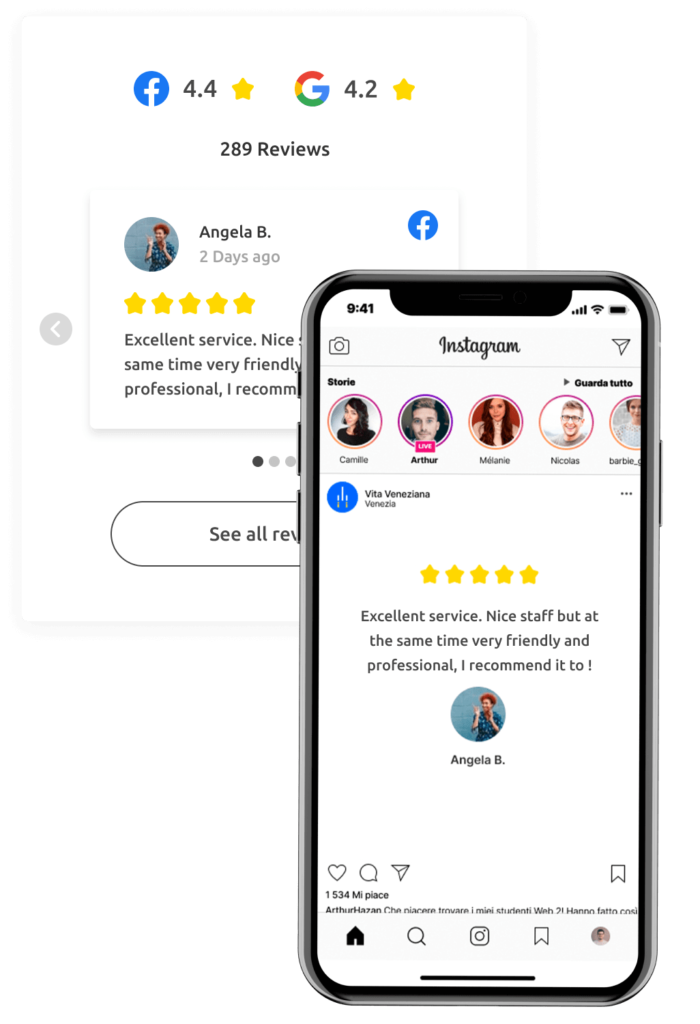
YOUR NEW REVIEW MANAGEMENT PLATFORM
STOP WASTING TIME TO MANAGE YOUR REVIEWS.
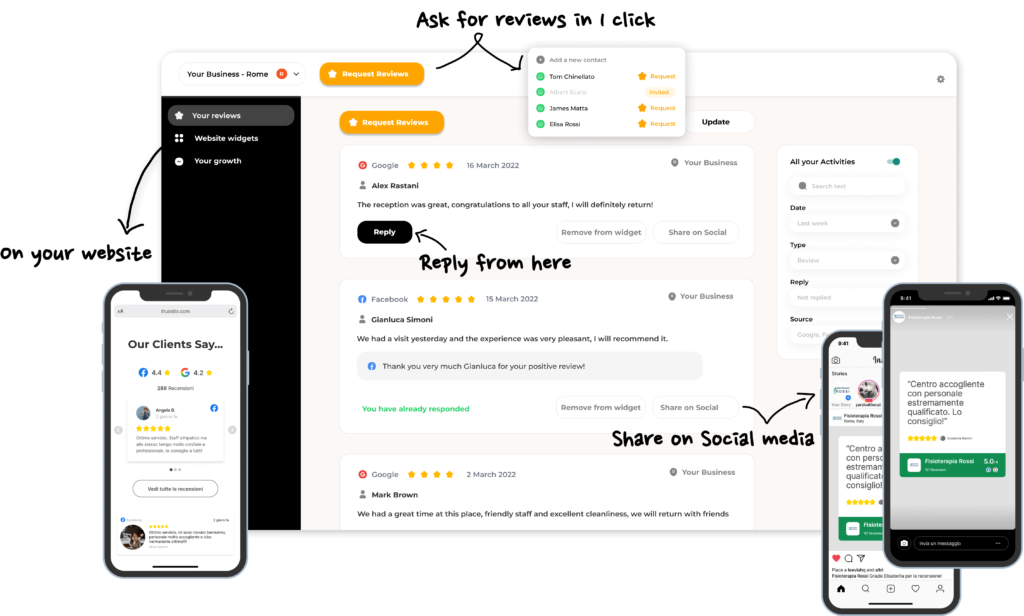